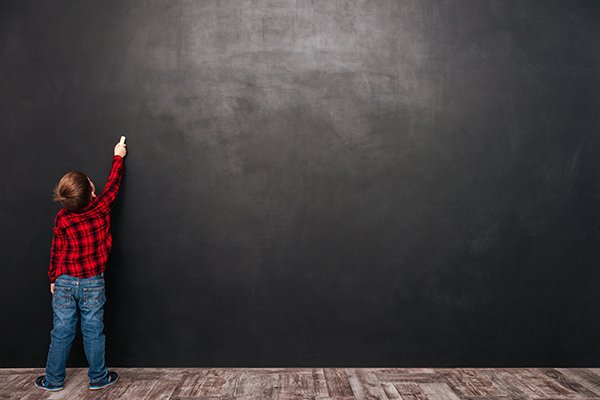
数组 Array
数组去重
functionnoRepeat(arr) {return[...newSet(arr)];}
查找数组最大
functionarrayMax(arr) {returnMath.max(...arr);}
查找数组最小
functionarrayMin(arr) {returnMath.min(...arr);}
返回已 size 为长度的数组分割的原数组
functionchunk(arr,size= 1) {returnArray.from({length: Math.ceil(arr.length /size),},(v, i) => arr.slice(i *size, i *size+size));}
检查数组中某元素出现的次数
functioncountOccurrences(arr, value) {returnarr.reduce((a, v) => (v === value ? a + 1 : a + 0), 0);}
扁平化数组
functionflatten(arr, depth = -1) {if (depth === -1) {return[].concat(...arr.map((v) => (Array.isArray(v) ? this.flatten(v) : v)));}if (depth === 1) {returnarr.reduce((a, v) => a.concat(v), []);}returnarr.reduce((a, v) => a.concat(Array.isArray(v) ? this.flatten(v, depth - 1) : v),[]);}
对比两个数组并且返回其中不同的元素
functiondiffrence(arrA, arrB) {returnarrA.filter((v) => !arrB.includes(v));}
返回两个数组中相同的元素
functionintersection(arr1, arr2) {returnarr2.filter((v) => arr1.includes(v));}
从右删除 n 个元素
functiondropRight(arr, n = 0) {returnn < arr.length ? arr.slice(0, arr.length - n) : [];}
截取第一个符合条件的元素及其以后的元素
functiondropElements(arr, fn) {while (arr.length && !fn(arr[0])) arr = arr.slice(1);returnarr;}
返回数组中下标间隔 nth 的元素
functioneveryNth(arr, nth) {returnarr.filter((v, i) => i % nth === nth - 1);}
返回数组中第 n 个元素
functionnthElement(arr, n = 0) {return(n >= 0 ? arr.slice(n, n + 1) : arr.slice(n))[0];}
返回数组头元素
functionhead(arr) {returnarr[0];}
返回数组末尾元素
functionlast(arr) {returnarr[arr.length - 1];}
数组乱排
functionshuffle(arr) {let array = arr;letindex= array.length;while (index) {index-= 1;let randomInedx = Math.floor(Math.random() *index);let middleware = array[index];array[index] = array[randomInedx];array[randomInedx] = middleware;}returnarray;}
浏览器对象 BOM
判读浏览器是否支持 CSS 属性
/*** 告知浏览器支持的指定css属性情况* @param {String}key- css属性,是属性的名字,不需要加前缀* @returns{String} - 支持的属性情况*/functionvalidateCssKey(key) {const jsKey = toCamelCase(key); // 有些css属性是连字符号形成if (jsKeyindocument.documentElement.style) {returnkey;}let validKey ="";// 属性名为前缀在js中的形式,属性值是前缀在css中的形式// 经尝试,Webkit 也可是首字母小写 webkitconst prefixMap = {Webkit:"-webkit-",Moz:"-moz-",ms:"-ms-",O:"-o-",};for(const jsPrefixinprefixMap) {const styleKey = toCamelCase(`${jsPrefix}-${jsKey}`);if (styleKeyindocument.documentElement.style) {validKey = prefixMap[jsPrefix] +key;break;}}returnvalidKey;}/*** 把有连字符号的字符串转化为驼峰命名法的字符串*/functiontoCamelCase(value) {returnvalue.replace(/-(\w)/g, (matched, letter) => {returnletter.toUpperCase();});}/*** 检查浏览器是否支持某个css属性值(es6版)* @param {String}key- 检查的属性值所属的css属性名* @param {String} value - 要检查的css属性值(不要带前缀)* @returns{String} - 返回浏览器支持的属性值*/functionvaliateCssValue(key, value) {const prefix = ["-o-","-ms-","-moz-","-webkit-",""];const prefixValue = prefix.map((item) => {returnitem + value;});const element = document.createElement("div");const eleStyle = element.style;// 应用每个前缀的情况,且最后也要应用上没有前缀的情况,看最后浏览器起效的何种情况// 这就是最好在prefix里的最后一个元素是''prefixValue.forEach((item) => {eleStyle[key] = item;});returneleStyle[key];}/*** 检查浏览器是否支持某个css属性值* @param {String}key- 检查的属性值所属的css属性名* @param {String} value - 要检查的css属性值(不要带前缀)* @returns{String} - 返回浏览器支持的属性值*/functionvaliateCssValue(key, value) {var prefix = ["-o-","-ms-","-moz-","-webkit-",""];var prefixValue = [];for(var i = 0; i < prefix.length; i++) {prefixValue.push(prefix[i] + value);}var element = document.createElement("div");var eleStyle = element.style;for(var j = 0; j < prefixvalue.length; j++) {eleStyle[key] = prefixValue[j];}returneleStyle[key];}functionvalidCss(key, value) {const validCss = validateCssKey(key);if (validCss) {returnvalidCss;}returnvaliateCssValue(key, value);}
返回当前网页地址
functioncurrentURL() {returnwindow.location.href;}
获取滚动条位置
functiongetScrollPosition(el = window) {return{x: el.pageXOffset !== undefined ? el.pageXOffset : el.scrollLeft,y: el.pageYOffset !== undefined ? el.pageYOffset : el.scrollTop,};}
获取 url 中的参数
functiongetURLParameters(url) {returnurl.match(/([^?=&]+)(=([^&]*))/g).reduce((a, v) => ((a[v.slice(0, v.indexOf("="))] = v.slice(v.indexOf("=") + 1)), a),{});}
页面跳转,是否记录在 history 中
functionredirect(url, asLink =true) {asLink ? (window.location.href = url) : window.location.replace(url);}
滚动条回到顶部动画
functionscrollToTop() {const scrollTop =document.documentElement.scrollTop || document.body.scrollTop;if (scrollTop > 0) {window.requestAnimationFrame(scrollToTop);window.scrollTo(0, c - c / 8);}else{window.cancelAnimationFrame(scrollToTop);}}
复制文本
functioncopy(str) {const el = document.createElement("textarea");el.value = str;el.setAttribute("readonly","");el.style.position ="absolute";el.style.left="-9999px";el.style.top="-9999px";document.body.appendChild(el);const selected =document.getSelection().rangeCount > 0? document.getSelection().getRangeAt(0):false;el.select();document.execCommand("copy");document.body.removeChild(el);if (selected) {document.getSelection().removeAllRanges();document.getSelection().addRange(selected);}}
检测设备类型
functiondetectDeviceType() {return/Android|webOS|iPhone|iPad|iPod|BlackBerry|IEMobile|Opera Mini/i.test(navigator.userAgent)?"Mobile":"Desktop";}
Cookie
增
functionsetCookie(key, value, expiredays) {var exdate = newDate();exdate.setDate(exdate.getDate() + expiredays);document.cookie =key+"="+escape(value) +(expiredays ==null?"":";expires="+ exdate.toGMTString());}
删
functiondelCookie(name) {var exp = newDate();exp.setTime(exp.getTime() - 1);var cval = getCookie(name);if (cval !=null) {document.cookie =name+"="+ cval +";expires="+ exp.toGMTString();}}
查
functiongetCookie(name) {var arr,reg = new RegExp("(^| )"+name+"=([^;]*)(;|$)");if ((arr = document.cookie.match(reg))) {returnarr[2];}else{returnnull;}}
日期 Date
时间戳转换为时间
默认为当前时间转换结果
isMs 为时间戳是否为毫秒
functiontimestampToTime(timestamp=Date.parse(newDate()), isMs =true) {constdate= newDate(timestamp* (isMs ? 1 : 1000));return`${date.getFullYear()}-${date.getMonth() + 1 < 10 ?"0"+ (date.getmonth() + 1) :date.getmonth() + 1}-${date.getDate()} ${date.getHours()}:${date.getMinutes()}:${date.getSeconds()}`;}
文档对象 DOM
固定滚动条
/*** 功能描述:一些业务场景,如弹框出现时,需要禁止页面滚动,这是兼容安卓和 iOS 禁止页面滚动的解决方案*/let scrollTop = 0;functionpreventScroll() {// 存储当前滚动位置scrollTop = window.scrollY;// 将可滚动区域固定定位,可滚动区域高度为 0 后就不能滚动了document.body.style["overflow-y"] ="hidden";document.body.style.position ="fixed";document.body.style.width ="100%";document.body.style.top= -scrollTop +"px";// document.body.style['overscroll-behavior'] ='none'}functionrecoverScroll() {document.body.style["overflow-y"] ="auto";document.body.style.position ="static";// document.querySelector('body').style['overscroll-behavior'] ='none'window.scrollTo(0, scrollTop);}
判断当前位置是否为页面底部
functionbottomVisible() {return(document.documentElement.clientHeight + window.scrollY >=(document.documentElement.scrollHeight ||document.documentElement.clientHeight));}
判断元素是否在可视范围内
functionelementIsVisibleInViewport(el, partiallyVisible =false) {const {top,left, bottom,right} = el.getBoundingClientRect();returnpartiallyVisible? ((top> 0 &&top< innerheight) ||(bottom > 0 && bottom < innerheight)) &&((left> 0 &&left< innerwidth) || (right> 0 &&right< innerwidth)):top>= 0 &&left>= 0 && bottom < ="innerHeight" &&right}
获取元素 css 样式
functiongetStyle(el, ruleName) {returngetComputedStyle(el,null).getPropertyValue(ruleName);}
进入全屏
functionlaunchFullscreen(element) {if (element.requestFullscreen) {element.requestFullscreen();}elseif (element.mozRequestFullScreen) {element.mozRequestFullScreen();}elseif (element.msRequestFullscreen) {element.msRequestFullscreen();}elseif (element.webkitRequestFullscreen) {element.webkitRequestFullScreen();}}launchFullscreen(document.documentElement);launchFullscreen(document.getElementById("id")); //某个元素进入全屏
退出全屏
functionexitFullscreen() {if (document.exitFullscreen) {document.exitFullscreen();}elseif (document.msExitFullscreen) {document.msExitFullscreen();}elseif (document.mozCancelFullScreen) {document.mozCancelFullScreen();}elseif (document.webkitExitFullscreen) {document.webkitExitFullscreen();}}exitFullscreen();
全屏事件
document.addEventListener("fullscreenchange",function(e) {if (document.fullscreenElement) {console.log("进入全屏");}else{console.log("退出全屏");}});
数字 Number
数字千分位分割
functioncommafy(num) {returnnum.toString().indexOf(".") !== -1? num.toLocaleString(): num.toString().replace(/(\d)(?=(?:\d{3})+$)/g,"$1,");}
生成随机数
functionrandomNum(min,max) {switch (arguments.length) {case1:returnparseInt(Math.random() *min+ 1, 10);case2:returnparseInt(Math.random() * (max-min+ 1) +min, 10);default:return0;}}